This is the start of — hopefully! — a new series for me, “TIL in front-end web development.” In case you have never been exposed to it before, “TIL” stands for “today I learned.” I believe the term has its origins on Reddit, that wretched hive of scum and and occasional hilarity.
My purpose with this series is to chronicle what I’m learning in my personal front-end development enrichment projects — which I suddenly have a lot more time for, thanks to unemployment. I’ve been doing this work for 10+ years professionally, but I still learn something new every day; every day I’m Googling something I don’t know, or some topic I need a refresher on.
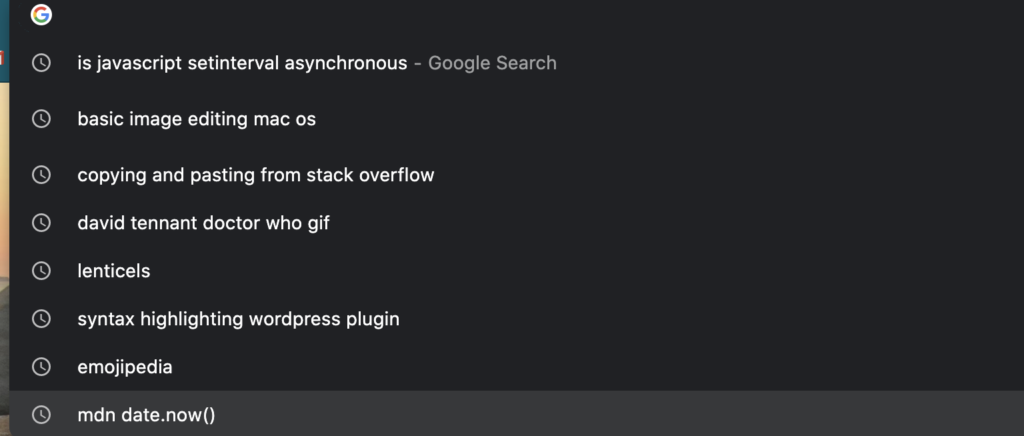
Without further ado, let’s talk about timers.
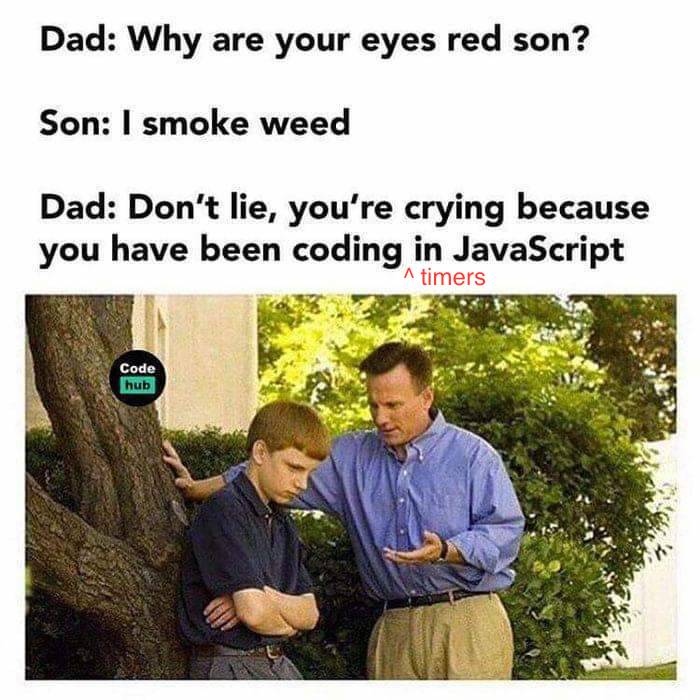
Brew tea as long as you want, as long as it’s five minutes
My most recent web development enrichment project has been Teamer, a tea timer webapp built using only HTML, CSS, and vanilla JS. Between that, and a code challenge I did for a job interview recently, I felt like I was thrown into the deep end of the “I write algorithms not event handlers” pool.
(“I write algorithms not event handlers” is clearly the next hit single from Panic! At the Disco).
Teamer started very simply, with just a series of buttons corresponding to each tea. When you clicked one, it would start a timer using the brewing time ceiling for that tea.
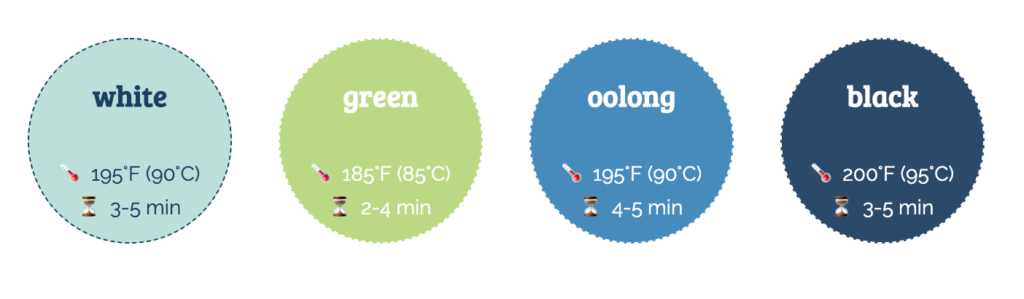
In this version, there was no possibility for adjustment. You wanted white tea, you got a five minute timer. That’s it. It wasn’t the most friendly user experience, but it was fine for a first pass.
(There are plenty of tutorials, Codepens, and JSFiddles out there about building a basic timer, but did I look at any of them? I did not. I knew I would learn it better if I had to figure it out myself).
The basic tea timing algorithm
Fundamentally, the timer algorithm is:
- Set the timer: set the visual display of the timer as minutes and seconds, and save it in milliseconds somewhere. (Where this value is saved varied between versions. Currently it’s stored as the
value
of the play/pause button). Logically enough, this is a function calledsetTimer()
; - Calculate the starting time in milliseconds. This uses the
Date.now()
method, which returns the current time in UTC/Unix epoch format, i.e. the number of milliseconds since 12:00am, January 1st, 1970. (Why 1970, I don’t know; I don’t make the rules. Maybe one day they’ll give me Tim Berners-Lee’s private number and I can call him up in the middle of the night and ask him questions like this). - Calculate the deadline — the point at which the timer will stop — by adding the milliseconds from step 1 onto the Unix start time from step 2.
- Start ticking: Using the
setInterval()
method, “tick” every second (1000ms) until the deadline. - On every tick: update the visual display as well as the current milliseconds, and compare the current milliseconds to the deadline .
Once I had that working predictably, I decided it was time to break everything add some user controls.
User controls = extra complication
(Matt saw me writing that title and said, “You’ll soon learn that users are the enemy”).
For the next iteration, I wanted to add a few new buttons: play/pause (that would allow you to pause and restart the timer), stop (that would stop the timer and set it to zero), and buttons to increment or decrement the timer by one minute or ten seconds.
That’s when things got complicated.
For one thing, I had to separate some of the logic from setTimer()
out into a new playTimer()
function. Previously, the timer started as soon as you selected a tea; now, I wanted the timer to start only when the user hit the play/pause button. So this necessitated some refactoring.
This is also where I created tick()
, breaking out an anonymous function inside setTimer()
/playTimer()
into a named function that I could run with setInterval()
. This function was designed to hold all the things that had to happen each time the timer ticked down — everything in step #5 above.
Using a combined play/pause button also meant that I had to keep track of the toggle state of the button, which I did with a playing
Boolean variable.
Incrementing/decrementing necessitated a probably-too-clever switch()
block based on the value
attribute on each increment/decrement button:
switch (parseInt(btn.value)) { case 60: deError(); setTimer(mins+1, secs); break; case -60: if (mins === 0) { setTimer(0, 0); } else setTimer(mins-1, secs); break; case 10: deError(); if (secs > 49) { setTimer(mins+1, (secs+10)-60); } else setTimer(mins, secs+10); break; case -10: if (secs < 10 && mins === 0) { setTimer(0, 0); } else if (secs < 10) { setTimer(mins-1, (secs-10)+60); } else setTimer(mins, secs-10); break; }
You can see that as well as resetting the timer, it handles some corner cases around converting from base 60 to base 10, i.e. when you reach 59, you need to roll over to zero, not 60. Also in there is logic to prevent you from setting the timer to negative numbers.
(I’m embarrassed to admit how long I swore at this switch()
statement, which seemed to be doing zilch on my first pass — no timers were being set. Then I realized that the btn.value
was coming in as a string, and thus it wouldn’t enter any of the cases — cases inside a switch()
block always have to be integers. JavaScript’s wibbly-wobbly typing strikes again!)
Feeling self-satisfied with my work, I pushed my code to my website and added it to Codepen. But then I noticed two other problems…
Take one down, pass it around… three gnarly Javascript bugs on the wall!
The two three problems are found were this:
- The timer was occasionally displaying as “:60” instead of “:00.”
- Adding time to a timer while it’s running causes the timer to become All Fucked Up (a technical term).
- Remember #1?…
The importance of being earnest (to the rounding method you choose at the start)
Debugging problem #1, I quickly realized it had nothing to do with the tortuous switch()
block — that was your first thought, too, eh?
However, I was concerned by the logic to calculate the remaining time on a timer — part of the tick()
function, called every 1000ms. It looked something like this:
if (end > now) {//time hasn't elapsed yet let diffMs = end - now; let diffMin = Math.floor(diffMs / 60000); let diffRemSec = Math.round(diffMs % 60000 / 1000 ); setTimer(diffMin, diffRemSec); }
(It actually wasn’t nearly as neat, because at this point I hadn’t yet figured out how to use the modulo operator to remove the minutes I had already accounted for. I highly recommend this Stack Overflow answer for the smarter way of doing things).
(Also no idea why I’m using let
for those three variables that I’m never reassigning. I guess I’m still not 100% used to the ES6 assignment keywords).
You may notice this disconnect between the Math.floor()
method I’m using for diffMin
and the Math.round()
method I’m using for diffRemSec
. Surely that may cause some weirdness, right? Plus there’s nothing stopping from Math.round()
from rounding up to 60; I’m not doing any base-conversion math like I am elsewhere. I also couldn’t remember why I had chosen Math.round()
instead of Math.floor()
— so, ultimately, I changed it to Math.floor()
.
Good news: I no longer saw “:60” instead of “:00”.
Bad news: the timer was now skipping numbers. (This is bug #3, alluded to above). Most noticeably, when you started the timer, it went straight from “:00” to “:58.” It would also occasionally skip numbers down the line.
I tried a bunch of different solutions for this. Applying one Math method, then another (no dice). Testing for that “:60” case and manually resetting it to “:00.” (That caused a slew of other issues). None of these really did what I needed them to do.
The basic problem was: it’s correct to use Math.floor()
, but the visual display of that data is incorrect.
Time is an illusion, and tea time doubly so.
Having ruled out rounding error, the conclusion I came to regarding the cause of the skips was: the code in tick()
took some non-zero amount of time to execute. Thus each measurement was being taken at 1000ms + 5? 10? ms, which over time would lead to drift.
Alternately — or in addition — there’s the fact that setInterval()
is cooperatively asynchronous. That means all synchronous code on the main thread must clear the call stack before the setInterval()
callback executes. (Hence the source of Every Hiring Manager’s Favorite JavaScript Question Ever).
Give that, I asked myself: can I run tick()
more frequently?
Before I dive into that, allow me a minor digression to talk about metaphors. (What can I say, I’m a word nerd as well as a nerd nerd).
The concept of a “tick” comes from an analog clock with hands, where each second is a movement of the hand of the clock, making a click or tick noise. If you speed up the ticks of an analog clock, you get a clock that runs fast; it will ultimately become so out of sync with Observed Time ™ that it becomes meaningless.
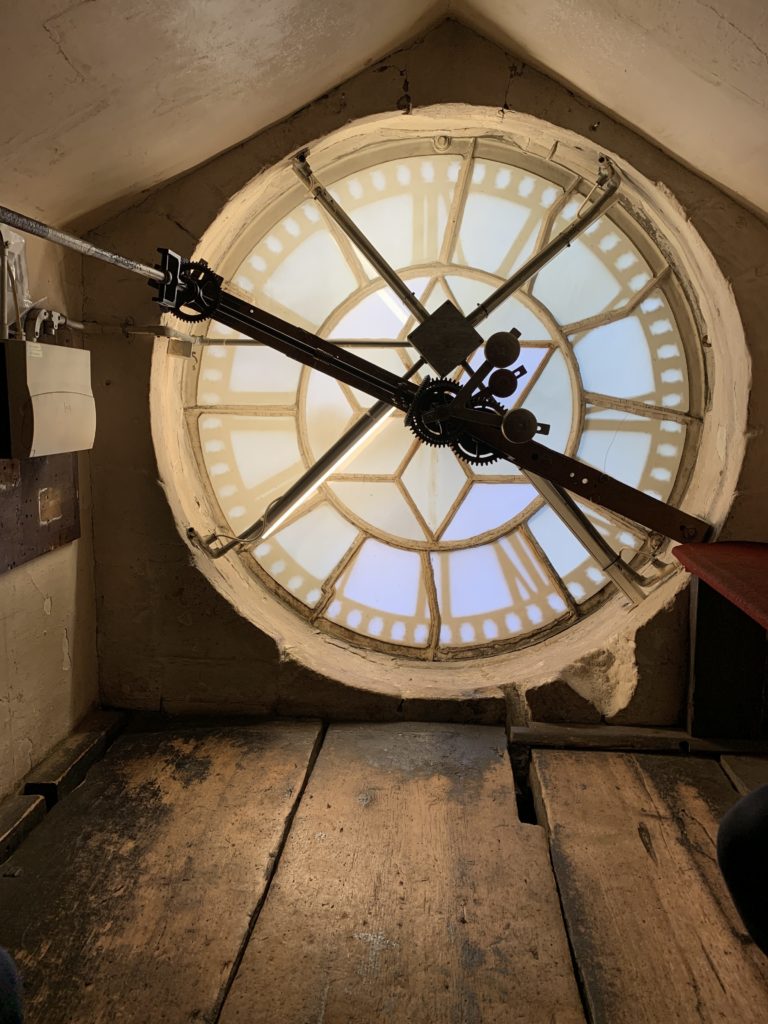
But that metaphor doesn’t transfer perfectly to our timer, does it? If you speed up the ticks, all that happens is the display is updated more often.
“Really, Lise?” Really. I know it doesn’t sound right, but bear in mind — what ultimately determines when the timer ends is the deadline in milliseconds. That never changes, no matter what you do on each tick. And both the deadline and the current milliseconds are grounded in Unix time, which is as official as it gets.
So what’s left? The visual display. There’s real math happening behind the face of this clock, so the updates will always be accurate to the current Unix time. But if the user sees skips in the sequence, they’re not going to trust that it’s accurate.
Here’s where I’m practicing my UX design skills. Because the conclusion I came to is:
- What’s most important to the user experience is the illusion that the timer is counting down at a rate of 1 second per second, with no gaps.
- The human brain can’t tell the difference between 1000ms and 990ms.
So that’s what I landed on — tick()
is run every 990ms instead of every 1000ms. Like so:
ticker = setInterval(tick, 990, deadline);
Relatedly, I want to take a moment to add: making setInterval()
a function expression in the global scope is crucial, so that you can use clearInterval()
to stop your timer. The assignment snippet above lives in playTimer()
, but it’s first declared near the top the JS file with let ticker
. (See, that’s the right usage of let
!)
Anyway, I tried a few different intervals, but I still saw skipping with 999ms, and with numbers below 990, I often saw flashes of the same number twice (as the display was updated with an identical number).
(You will see those once in a blue moon even with 990ms, but it’s not super noticeable. If I decide to add a CSS transition to the numbers, I might have to account for that — maybe setting up a control block that only updates the number if it’s different from the immediately previous number?)
Once again, JavaScript allows me to pretend I’m a Time Lord.
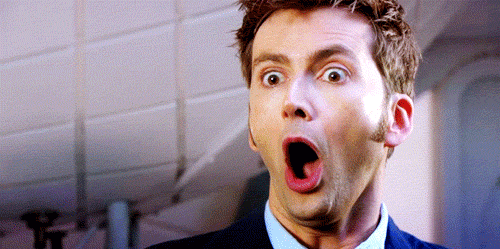
Who does number two work for?
I didn’t forget bug #2 (inc/decrementing a timer while it’s running causes weirdness), I promise! Although I did set it aside temporarily while I dealt with JavaScript’s timey-wimeyness.
I found there were a couple of things going on here:
Problem the first: the deadline was not being updated to reflect the new deadline. When you added/removed time without going through the click handler on the play/pause, you were never invoking playTimer()
, and thus never setting a new deadline. So if you added a minute to a 3-minute timer, it would look like a 4 minute timer, but in the code it was still waiting for a time three minutes in the future.
So, I should explicitly invoke playTimer()
after my big ol’ switch()
block, in the click handler for the increment/decrement buttons, right?
But hold on, what if the timer is already paused? Then I’d be doing it twice; once at the end of the block and once when the play/pause button is clicked. I definitely don’t want to set the deadline or change the playing
state twice.
So this is was my first iteration:
if (playing === true) { playTimer(); //if timer is running, run playTimer() to update the deadline; otherwise it will update when the play/pause button is clicked again }
That fixed the “resetting the deadline” issue, but in classic debugging fashion, raised a new problem: now the timer would flash between the old time and the new time. Wtf?
I eventually figured out I had to stop the ticker (with clearInterval()
, in the stopTicker()
function) before starting another one. I’m guessing this is because every time you run playTimer()
, you create a new instance of ticker
; they each have their own closure with the same starting values, but then they diverge based on the differences in the environment at the times they’re run. You get the flashing behavior because the two different tickers are trying to modify the same DOM element, like two children fighting over a toy.
… but my understanding of the inner working of JavaScript and closures is imperfect, so take this analysis with a grain of salt.
Anywho! This worked fine:
if (playing === true) { stopTicker(); playTimer(); //if timer is running, run playTimer() to update the deadline; otherwise it will update when the play/pause button is clicked again }
In Conclusion: What I Learned
I’ve finished all the time juggling for my tea timer app (for now, she says, ominously), and now I’m working on adding more features. An alarm when the timer ends? Using localstorage
to allow users to add custom teas? Heck, maybe I’ll even add the ability to have multiple timers running at once, as an excuse to work with classes in JavaScript.
But to summarize what I learned about timers in the process of writing Teamer:
- Date/time math is easier with modulo.
- User control makes everything more complicated.
- Sometimes the answer to “why doesn’t this razzlefrazzle work?” is “you need to parse it as an integer.”
- Be consistent in your rounding methods, and always use
Math.floor()
when working with time. - If you want to stop your
setInterval()
, you need to tie it to a function expression in the global scope. - Just because your timer is meant to update every second, doesn’t mean it needs to update at precisely that interval — the illusion is more important than the truth.
- Honestly, timers are a solved problem, and if I were doing this for any purpose other than my own edification, I’d probably go with the time-tested approach of copying and pasting from Stack Overflow.
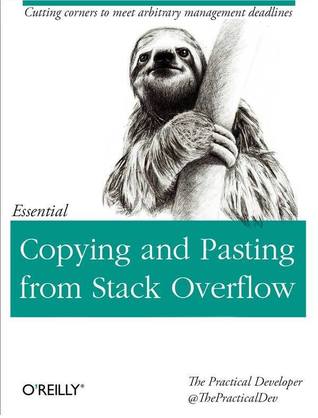
- I still maintain that working with JavaScript is like being a Time Lord — you get to work with a lot of wibbly-wobbly timey-wimey… stuff.
- I wanna meet someone who was born at midnight on January 1st, 1970, just so I can give them the nickname “UTC.”
If you want to see the current iteration of the code with highlighting ‘n stuff, it’s up on Codepen.
And that is already way longer than I ever intended this post to be. Hopefully it is useful to someone other than future!Lise.